本文介绍: 一个普通的窗体应用,6个buttonusing System;using System.Collections.Generic;using System.ComponentModel;using System.Data;using System.Drawing;using System.Linq;using System.Text;using System.Threading.Tasks;using System.Windows.Forms;using MsWord = Mic…
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
using MsWord = Microsoft.Office.Interop.Word;
using Microsoft.Office.Interop.Word;
using System.IO;
using Spire.Doc;
using Spire.Doc.Documents;
using Spire.Doc.Fields;
namespace wordcomtest
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void Form1_Load(object sender, EventArgs e)
{
}
public static class common
{
public static MsWord.Application oWordApplic;//a reference to Wordapplication
public static MsWord.Document oDoc;//a reference to thedocument
public static string doc_file_name = Directory.GetCurrentDirectory() + @"content.doc";
}
private void button1_Click(object sender, EventArgs e)
{
try
{
if (File.Exists(common.doc_file_name))
{
File.Delete(common.doc_file_name);
}
common.oWordApplic = new MsWord.Application();
object missing = System.Reflection.Missing.Value;
}
catch (Exception e2)
{
MessageBox.Show(e2.Message);
}
try
{ }
catch (Exception e2)
{
MessageBox.Show(e2.Message);
}
}
private void button2_Click(object sender, EventArgs e)
{
try
{
MsWord.Range curRange;
object curTxt;
int curSectionNum = 1;
common.oDoc = common.oWordApplic.Documents.Add();
common.oDoc.Activate();
//Console.WriteLine(" 正在生成文档小节");
object section_nextPage = MsWord.WdBreakType.wdSectionBreakNextPage;
object page_break = MsWord.WdBreakType.wdPageBreak;
//添加三个分节符,共四个小节
for (int si = 0; si < 2; si++)
{
common.oDoc.Paragraphs[1].Range.InsertParagraphAfter();
common.oDoc.Paragraphs[1].Range.InsertBreak(ref section_nextPage);
}
}
catch (Exception e2)
{
MessageBox.Show(e2.Message);
}
}
private void button3_Click(object sender, EventArgs e)
{
try
{
int curSectionNum = 1;
var curRange = common.oDoc.Sections[curSectionNum].Range.Paragraphs[1].Range;
curRange.Select();
string one_str, key_word;
//摘要的文本来自 abstract.txt 文本文件
StreamReader file_abstract = new StreamReader("abstract.txt");
common.oWordApplic.Options.Overtype = false;//overtype 改写模式
MsWord.Selection currentSelection = common.oWordApplic.Selection;
if (currentSelection.Type == MsWord.WdSelectionType.wdSelectionNormal)
{
one_str = file_abstract.ReadLine();//读入题目
currentSelection.TypeText(one_str);
currentSelection.TypeParagraph(); //添加段落标记
currentSelection.TypeText(" 摘要");//写入" 摘要" 二字
currentSelection.TypeParagraph(); //添加段落标记
key_word = file_abstract.ReadLine();//读入题目
one_str = file_abstract.ReadLine();//读入段落文本
while (one_str != null)
{
currentSelection.TypeText(one_str);
currentSelection.TypeParagraph(); //添加段落标记
one_str = file_abstract.ReadLine();
}
currentSelection.TypeText(" 关键字:");
currentSelection.TypeText(key_word);
currentSelection.TypeParagraph(); //添加段落标记
}
file_abstract.Close();
}
catch (Exception e2)
{
MessageBox.Show(e2.Message);
}
}
private void button4_Click(object sender, EventArgs e)
{
try
{
int curSectionNum = 3;
common.oDoc.Sections[curSectionNum].Range.Paragraphs[1].Range.Select();
Range curRange = common.oDoc.Sections[curSectionNum].Range.Paragraphs[1].Range;
Console.WriteLine(" 正在设置标题样式");
object wdFontSizeIndex;
wdFontSizeIndex = 14;//此序号在 word 中的编号是格式 > 显示格式 > 样式和格式 > 显示
//14 即是标题一一级标题:三号黑体。
common.oWordApplic.ActiveDocument.Styles.get_Item(ref wdFontSizeIndex).ParagraphFormat.Alignment =
MsWord.WdParagraphAlignment.wdAlignParagraphCenter;
common.oWordApplic.ActiveDocument.Styles.get_Item(ref wdFontSizeIndex).Font.Name = " 黑体";
common.oWordApplic.ActiveDocument.Styles.get_Item(ref wdFontSizeIndex).Font.Size = 16;//三号
wdFontSizeIndex = 15;//15 即是标题二二级标题:小三号黑体。
common.oWordApplic.ActiveDocument.Styles.get_Item(ref wdFontSizeIndex).Font.Name = " 黑体";
common.oWordApplic.ActiveDocument.Styles.get_Item(ref wdFontSizeIndex).Font.Size = 15;//小三
//用指定的标题来设定文本格式
object Style1 = MsWord.WdBuiltinStyle.wdStyleHeading1;//一级标题:三号黑体。
object Style2 = MsWord.WdBuiltinStyle.wdStyleHeading2;//二级标题:小三号黑体。
common.oDoc.Sections[curSectionNum].Range.Select();
var currentSelection = common.oWordApplic.Selection;
//读入第一章文本信息
StreamReader file_content = new StreamReader("content.txt");
var one_str = file_content.ReadLine();//一级标题
currentSelection.TypeText(one_str);
currentSelection.TypeParagraph(); //添加段落标记
one_str = file_content.ReadLine();//二级标题
currentSelection.TypeText(one_str);
currentSelection.TypeParagraph(); //添加段落标记
one_str = file_content.ReadLine();//正文
while (one_str != null)
{
currentSelection.TypeText(one_str);
currentSelection.TypeParagraph(); //添加段落标记
one_str = file_content.ReadLine();//正文
}
file_content.Close();
//段落的对齐方式
curRange = common.oDoc.Sections[curSectionNum].Range.Paragraphs[1].Range;
curRange.set_Style(ref Style1);
common.oDoc.Sections[curSectionNum].Range.Paragraphs[1].Alignment =
MsWord.WdParagraphAlignment.wdAlignParagraphCenter;
curRange = common.oDoc.Sections[curSectionNum].Range.Paragraphs[2].Range;
curRange.set_Style(ref Style2);
//第一章正文文本格式
for (int i = 3; i < common.oDoc.Sections[curSectionNum].Range.Paragraphs.Count; i++)
{
curRange = common.oDoc.Sections[curSectionNum].Range.Paragraphs[i].Range;
curRange.Select();
curRange.Font.Name = " 宋体";
curRange.Font.Size = 12;
common.oDoc.Sections[curSectionNum].Range.Paragraphs[i].LineSpacingRule =
MsWord.WdLineSpacing.wdLineSpaceMultiple;
//多倍行距,1.25 倍,这里的浮点值是以 point 为单位的,不是行距的倍数
common.oDoc.Sections[curSectionNum].Range.Paragraphs[i].LineSpacing = 15f;
common.oDoc.Sections[curSectionNum].Range.Paragraphs[i].IndentFirstLineCharWidth(2);
}
}
catch (Exception e2)
{
MessageBox.Show(e2.Message);
}
}
private void button5_Click(object sender, EventArgs e)
{
try
{
object missing = System.Reflection.Missing.Value;
//设置页脚 section 1 摘要
int curSectionNum = 1;
common.oDoc.Sections[curSectionNum].Range.Select();
//进入页脚视图
common.oWordApplic.ActiveWindow.ActivePane.View.SeekView =
MsWord.WdSeekView.wdSeekCurrentPageFooter;
common.oDoc.Sections[curSectionNum].
Headers[MsWord.WdHeaderFooterIndex.wdHeaderFooterPrimary].
Range.Borders[MsWord.WdBorderType.wdBorderBottom].LineStyle =
MsWord.WdLineStyle.wdLineStyleNone;
common.oWordApplic.Selection.HeaderFooter.PageNumbers.RestartNumberingAtSection = true;
common.oWordApplic.Selection.HeaderFooter.PageNumbers.NumberStyle
= MsWord.WdPageNumberStyle.wdPageNumberStyleUppercaseRoman;
common.oWordApplic.Selection.HeaderFooter.PageNumbers.StartingNumber = 1;
//切换到文档
common.oWordApplic.ActiveWindow.ActivePane.View.SeekView =
MsWord.WdSeekView.wdSeekMainDocument;
//Console.WriteLine(" 正在设置第二节目录页眉内容");
//设置页脚 section 2 目录
curSectionNum = 2;
common.oDoc.Sections[curSectionNum].Range.Select();
//进入页脚视图
common.oWordApplic.ActiveWindow.ActivePane.View.SeekView =
MsWord.WdSeekView.wdSeekCurrentPageFooter;
common.oDoc.Sections[curSectionNum].
Headers[MsWord.WdHeaderFooterIndex.wdHeaderFooterPrimary].
Range.Borders[MsWord.WdBorderType.wdBorderBottom].LineStyle =
MsWord.WdLineStyle.wdLineStyleNone;
common.oWordApplic.Selection.HeaderFooter.PageNumbers.RestartNumberingAtSection = false;
common.oWordApplic.Selection.HeaderFooter.PageNumbers.NumberStyle
= MsWord.WdPageNumberStyle.wdPageNumberStyleUppercaseRoman;
//oWordApplic.Selection.HeaderFooter.PageNumbers.StartingNumber = 1;
//切换到文档
common.oWordApplic.ActiveWindow.ActivePane.View.SeekView =
MsWord.WdSeekView.wdSeekMainDocument;
//第一章页眉页码设置
curSectionNum = 3;
common.oDoc.Sections[curSectionNum].Range.Select();
//切换入页脚视图
common.oWordApplic.ActiveWindow.ActivePane.View.SeekView =
MsWord.WdSeekView.wdSeekCurrentPageFooter;
var currentSelection = common.oWordApplic.Selection;
var curRange = currentSelection.Range;
//本节页码不续上节
common.oWordApplic.Selection.HeaderFooter.PageNumbers.RestartNumberingAtSection = true;
//页码格式为阿拉伯
common.oWordApplic.Selection.HeaderFooter.PageNumbers.NumberStyle
= MsWord.WdPageNumberStyle.wdPageNumberStyleArabic;
//起如页码为 1
common.oWordApplic.Selection.HeaderFooter.PageNumbers.StartingNumber = 1;
//添加页码域
object fieldpage = MsWord.WdFieldType.wdFieldPage;
common.oWordApplic.Selection.Fields.Add(common.oWordApplic.Selection.Range,
ref fieldpage, ref missing, ref missing);
//居中对齐
common.oWordApplic.Selection.ParagraphFormat.Alignment =
MsWord.WdParagraphAlignment.wdAlignParagraphCenter;
//本小节不链接到上一节
common.oDoc.Sections[curSectionNum].Headers[Microsoft.Office.Interop.
Word.WdHeaderFooterIndex.wdHeaderFooterPrimary].LinkToPrevious = false;
//切换入正文视图
common.oWordApplic.ActiveWindow.ActivePane.View.SeekView =
MsWord.WdSeekView.wdSeekMainDocument;
}
catch (Exception e2)
{
MessageBox.Show(e2.Message);
}
}
private void button6_Click(object sender, EventArgs e)
{
try
{
//common.oDoc.Fields[1].Update();
//保存文档
//Console.WriteLine(" 正在保存 Word 文档");
//string doc_file_name = Directory.GetCurrentDirectory() + @"content.doc";
object fileName;
fileName = common.doc_file_name;
common.oDoc.SaveAs(ref fileName);
common.oDoc.Close();
//释放 COM 资源
System.Runtime.InteropServices.Marshal.ReleaseComObject(common.oDoc);
common.oDoc = null;
common.oWordApplic.Quit();
System.Runtime.InteropServices.Marshal.ReleaseComObject(common.oWordApplic);
common.oWordApplic = null;
Spire.Doc.Document document = new Spire.Doc.Document(@"content.doc");
Spire.Doc.Section section = document.AddSection();
Spire.Doc.HeaderFooter footer = document.Sections[0].HeadersFooters.Footer;
//Add text and image to the footer
Spire.Doc.Documents.Paragraph paragraph = footer.AddParagraph();
DocPicture footerImage = paragraph.AppendPicture(Image.FromFile("whu.png"));
TextRange TR = paragraph.AppendText("Supported and Hosted by the non-profit Wikimedia Foundation.");
//Format the text and image
paragraph.Format.HorizontalAlignment = Spire.Doc.Documents.HorizontalAlignment.Left;
TR.CharacterFormat.FontName = "Calibri";
TR.CharacterFormat.Bold = true;
//添加一个Shape,并设置其大小和样式
Spire.Doc.Documents.Paragraph paragraph1 = section.AddParagraph();
ShapeObject shape = paragraph1.AppendShape(240, 60, ShapeType.TextWave);
//设置shape的位置
shape.VerticalPosition = 80;
shape.HorizontalPosition = 100;
//写入艺术字文本和设置斜体
shape.WordArt.Text = "艺术字效果";
shape.WordArt.Italic = true;
//设置文字填充样式
shape.FillColor = System.Drawing.Color.Red;
shape.StrokeColor = System.Drawing.Color.Gray;
// Save the document and launch to see the output
Spire.Doc.Documents.Paragraph paragraph2 = document.Sections[0].Paragraphs[2];
Spire.Doc.Fields.Footnote footnote = paragraph2.AppendFootnote(FootnoteType.Footnote);
DocumentObject obj = null;
for (int i = 0; i < paragraph.ChildObjects.Count; i++)
{
obj = paragraph.ChildObjects[i];
if (obj.DocumentObjectType == DocumentObjectType.TextRange)
{
TextRange textRange = obj as TextRange;
if (textRange.Text == "推箱子")
{
//为添加脚注的字符串设置加粗格式
textRange.CharacterFormat.Bold = true;
//插入脚注
paragraph.ChildObjects.Insert(i + 1, footnote);
break;
}
}
}
TextRange text = footnote.TextBody.AddParagraph().AppendText("推箱子是一款来自日本的古老游戏,其设计目的是训练人的逻辑思维能力。游戏场景一般是设定在空间狭小的仓库中,要求把箱子摆放到指定位置。这就要求玩家巧妙的运用有限的空间和通道,合理的安排箱子的位置和移动次序才可能完成任务。");
text.CharacterFormat.FontName = "Arial Black";
text.CharacterFormat.FontSize = 9;
text.CharacterFormat.TextColor = Color.DarkGray;
footnote.MarkerCharacterFormat.FontName = "Calibri";
footnote.MarkerCharacterFormat.FontSize = 12;
footnote.MarkerCharacterFormat.Bold = true;
footnote.MarkerCharacterFormat.TextColor = Color.DarkGreen;
document.SaveToFile("content.doc", FileFormat.Doc);
//System.Diagnostics.Process.Start("text.docx");
}
catch (Exception e2)
{
MessageBox.Show(e2.Message);
}
}
}
}
添加com引用
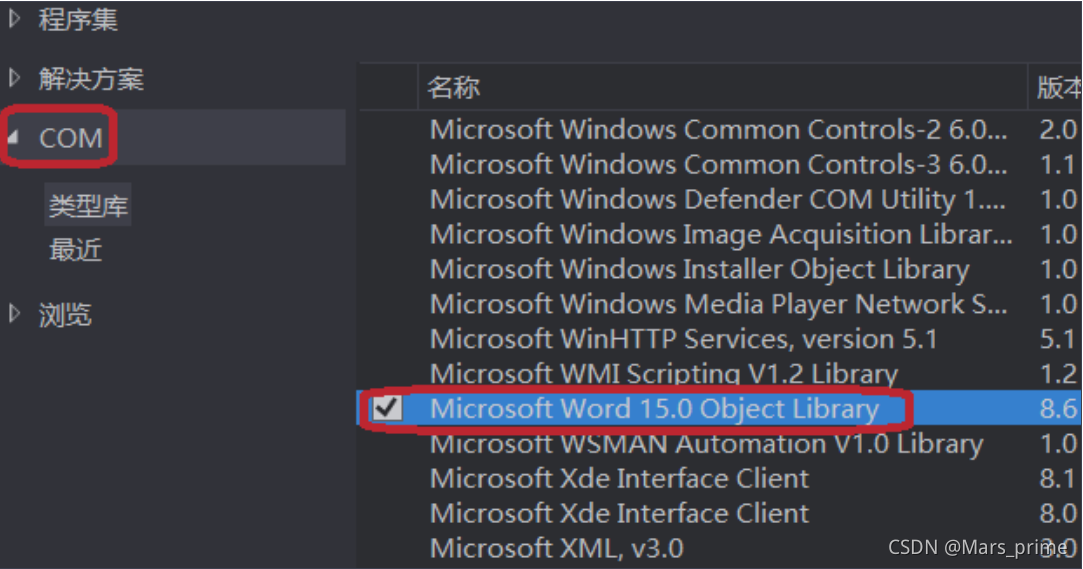
在程序代码源文件中添加命名空间支持:
using MsWord=Microsoft.Office.Interop.Word;
MsWord.Application oWordApplic;//a reference to Wordapplication
{
}
{
MessageBox.Show(e2.Message);
}
检测到旧的 word 文 档后删除旧文档。
string doc_file_name = Directory.GetCurrentDirectory() + @"content.doc";
if (File.Exists(doc_file_name))
{
File.Delete(doc_file_name);
}
oWordApplic = new MsWord.Application();
object missing = System.Reflection.Missing.Value;
创建 Word 文档的小节
MsWord.Range curRange;
object curTxt;
int curSectionNum = 1;
oDoc = oWordApplic.Documents.Add(ref missing, ref missing, ref missing, ref missing);
oDoc.Activate();
Console.WriteLine(" 正在生成文档小节");
object section_nextPage = MsWord.WdBreakType.wdSectionBreakNextPage;
object page_break = MsWord.WdBreakType.wdPageBreak;
//添加三个分节符,共四个小节
for (int si = 0; si < 4; si++)
{
oDoc.Paragraphs[1].Range.InsertParagraphAfter();
oDoc.Paragraphs[1].Range.InsertBreak(ref section_nextPage);
}
原文地址:https://blog.csdn.net/Mars_prime/article/details/120943681
本文来自互联网用户投稿,该文观点仅代表作者本人,不代表本站立场。本站仅提供信息存储空间服务,不拥有所有权,不承担相关法律责任。
如若转载,请注明出处:http://www.7code.cn/show_20508.html
如若内容造成侵权/违法违规/事实不符,请联系代码007邮箱:suwngjj01@126.com进行投诉反馈,一经查实,立即删除!
声明:本站所有文章,如无特殊说明或标注,均为本站原创发布。任何个人或组织,在未征得本站同意时,禁止复制、盗用、采集、发布本站内容到任何网站、书籍等各类媒体平台。如若本站内容侵犯了原著者的合法权益,可联系我们进行处理。