一:Python调用C语言场景
1,已经写好的C语言代码,不容易用Python实现,想直接通过Python调用写好的C语言代码
2,C比Python快(只是从语言层面,不能绝对说C程序就是比Python快)
3,想直接调用C语言丰富的库
二:Python的CTypes库
1.SWIG,编写一个额外的接口文件来作为SWIG(终端工具)的入口
但是 CTypes是最简单上手的一种,本文将用CTypes来演示如何在Python中调用C
>>> import ctypes
>>>
>>> dir(ctypes)
[‘ARRAY’, ‘ArgumentError‘, ‘Array’, ‘BigEndianStructure‘, ‘CDLL’, ‘CFUNCTYPE’, ‘DEFAULT_MODE’, ‘LibraryLoader‘, ‘LittleEndianStructure‘, ‘POINTER’, ‘PYFUNCTYPE’, ‘PyDLL’, ‘RTLD_GLOBAL’, ‘RTLD_LOCAL’, ‘SetPointerType’, ‘Structure‘, ‘Union‘, ‘_CFuncPtr‘, ‘_FUNCFLAG_CDECL’, ‘_FUNCFLAG_PYTHONAPI’, ‘_FUNCFLAG_USE_ERRNO’, ‘_FUNCFLAG_USE_LASTERROR’, ‘_Pointer’, ‘_SimpleCData’, ‘__builtins__‘, ‘__cached__‘, ‘__doc__‘, ‘__file__‘, ‘__loader__’, ‘__name__’, ‘__package__’, ‘__path__’, ‘__spec__’, ‘__version__’, ‘_c_functype_cache‘, ‘_calcsize‘, ‘_cast‘, ‘_cast_addr‘, ‘_check_size‘, ‘_ctypes_version‘, ‘_dlopen‘, ‘_endian’, ‘_memmove_addr‘, ‘_memset_addr‘, ‘_os‘, ‘_pointer_type_cache‘, ‘_reset_cache‘, ‘_string_at’, ‘_string_at_addr‘, ‘_sys‘, ‘_wstring_at’, ‘_wstring_at_addr‘, ‘addressof’, ‘alignment’, ‘byref‘, ‘c_bool‘, ‘c_buffer‘, ‘c_byte‘, ‘c_char‘, ‘c_char_p‘, ‘c_double‘, ‘c_float‘, ‘c_int‘, ‘c_int16′, ‘c_int32′, ‘c_int64′, ‘c_int8′, ‘c_long‘, ‘c_longdouble‘, ‘c_longlong‘, ‘c_short‘, ‘c_size_t’, ‘c_ssize_t’, ‘c_ubyte‘, ‘c_uint‘, ‘c_uint16′, ‘c_uint32′, ‘c_uint64′, ‘c_uint8′, ‘c_ulong‘, ‘c_ulonglong‘, ‘c_ushort‘, ‘c_void_p‘, ‘c_voidp‘, ‘c_wchar‘, ‘c_wchar_p‘, ‘cast‘, ‘cdll‘, ‘create_string_buffer‘, ‘create_unicode_buffer‘, ‘get_errno‘, ‘memmove’, ‘memset‘, ‘pointer‘, ‘py_object‘, ‘pydll‘, ‘pythonapi‘, ‘resize‘, ‘set_errno‘, ‘sizeof‘, ‘string_at’, ‘wstring_at’]
>>>
其中cdll类对于我们接下来调用C非常有用处,我们通过help来查看cdll类,可以看到可以通过LoadLibrary来加载C的库(在Linux中是.so,在windows中是.dll)
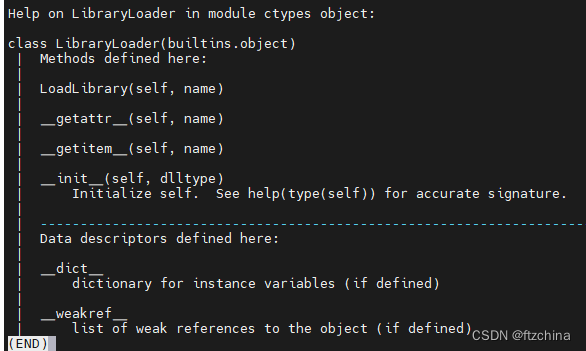
三:实战
1,调用C语言的函数
#include<stdio.h>
void cprintf()
{
printf("Hello word!n");
return;
}
int add(int a, int b)
{
return a+b;
}
int cscanf()
{
int a;
int b;
scanf("%d %d",&a,&b);
return a+b;
}
三个函数,一个是有打印函数,一个是有传参函数,一个是有输入函数,将C编译成动态库
gcc -fPIC -c ctest.c -o ctest.o
gcc -shared -o libctest.so ctest.o
然后我们在Python中调用这三个函数,
from ctypes import *
cLib = cdll.LoadLibrary('./libctest.so')
cLib.cprintf()
a = 1
b = 2
sum = cLib.add(a,b)
print(f'{a}+{b}={sum}')
cSum = cLib.cscanf()
print(f'cSum={cSum}')
2,调用C语言返回值是指针的函数
C代码
#include<stdio.h>
#include<stdlib.h>
#include<string.h>
#define uint8_t unsigned char
#define uint16_t unsigned short
typedef struct TagMyStruct
{
char name[10];
uint8_t age;
int score;
} MyStruct,*MyStructPointer;
MyStructPointer cstruct_get_data_pointer() // 返回结构体指针
{
MyStructPointer pt = (MyStructPointer)malloc(sizeof(MyStructPointer));
strcpy(pt->name, "ftz");
pt->age = 18;
pt->score = 88;
return pt;
}
MyStruct cstruct_get_data_self() // 返回结构体
{
return (MyStruct){"csdn",100,99};
}
python调用
from ctypes import *
cLib = cdll.LoadLibrary('./cstruct.so')
class MyStruct(Structure):
_fields_ = [
('name', c_char*10),
('age', c_ubyte),
('score', c_int),
]
cLib.cstruct_get_data_self.restype = MyStruct #指定函数返回值结构体本身
dat = cLib.cstruct_get_data_self()
print("=====get struct self from .so function=======")
print("name=",dat.name)
print("age=",dat.age)
print("score=",dat.score)
cLib.cstruct_get_data_pointer.restype = POINTER(MyStruct) #指定函数返回值结构体指针
dat = cLib.cstruct_get_data_pointer()
print("=====get struct point from .so function=======")
print("name=",dat.contents.name)
print("age=",dat.contents.age)
print("score=",dat.contents.score)
原文地址:https://blog.csdn.net/qq_27071221/article/details/134768391
本文来自互联网用户投稿,该文观点仅代表作者本人,不代表本站立场。本站仅提供信息存储空间服务,不拥有所有权,不承担相关法律责任。
如若转载,请注明出处:http://www.7code.cn/show_34318.html
如若内容造成侵权/违法违规/事实不符,请联系代码007邮箱:suwngjj01@126.com进行投诉反馈,一经查实,立即删除!